Programming
Here you can quickly jump to a particular “CONTENT” related to the Topic: “PROGRAMMING”.
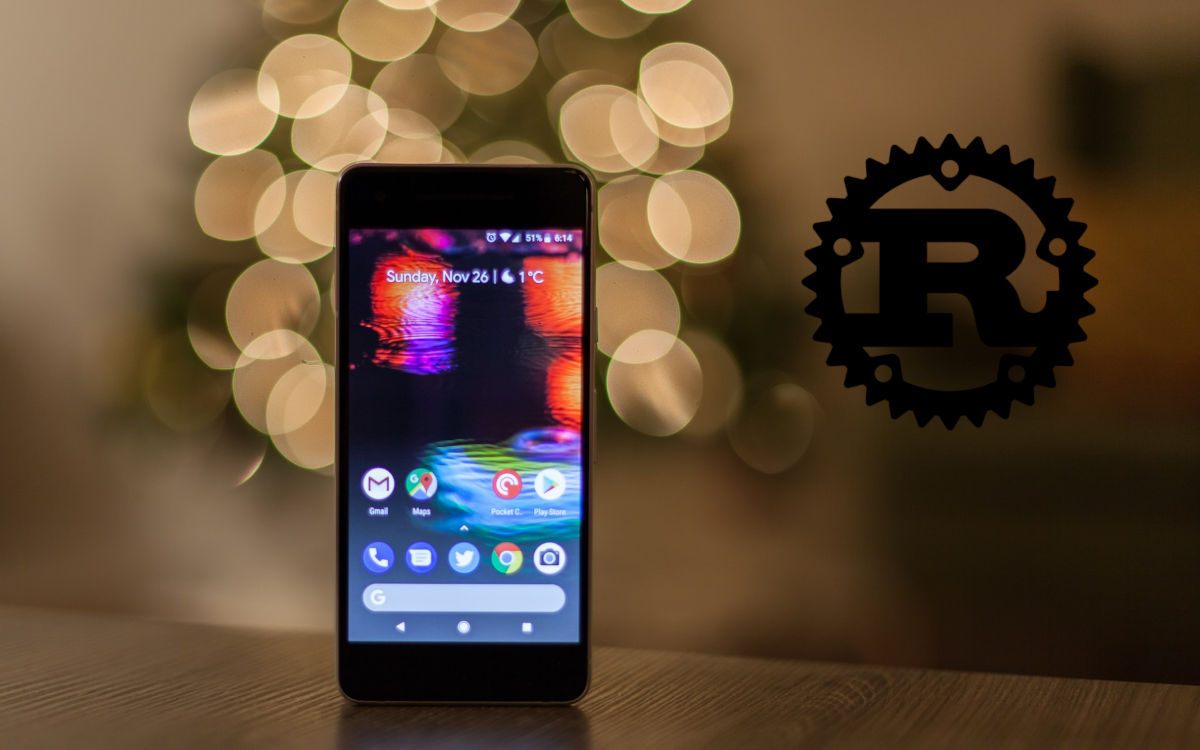
Rust cross-platform... The Android part...
In this journey, we will go through the process, infrastructure and architecture on how we can integrate Rust in our Android Project in a cross-platform development way. Topics like JNI and NDK are also covered, plus some anti-patterns and real use cases/scenarios. Continue reading Rust cross-platform... The Android part...
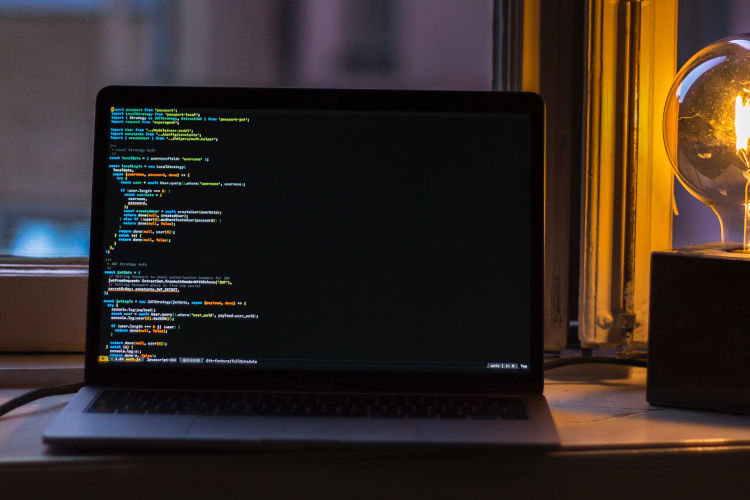
Cooking Effective Code Reviews.
Reviewing code (PRs) is not an easy task, so in this post I will share tips and tricks on how your code reviews can better contribute to code quality, be more effective and increase team morale by following a structured and organized process. Let’s jump in! Continue reading Cooking Effective Code Reviews.
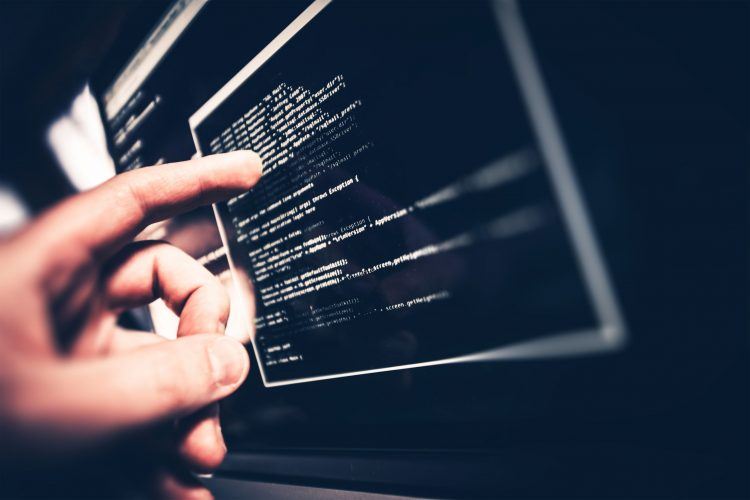
Technical Debt... GURU LEVEL UNLOCKED!
As Software Engineers we know that Technical Debt and Legacy Code are familiar concepts we have to live with. Code healthiness and maintenance are challenging, so let’s dive into tips and techniques on how to effectively address this problem. Continue reading Technical Debt... GURU LEVEL UNLOCKED!
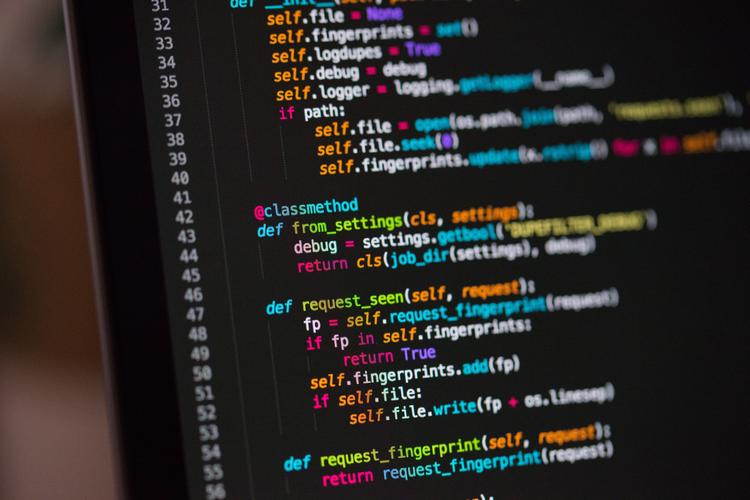
Architecting Android...Reloaded.
Android Architecture has been evolving over the years and we need to adapt to the current times. Here we will dive into Functional Programming, OOP, Error Handling, Modularization and Patterns for the Android Platform, and everything written in Kotlin. Continue reading Architecting Android...Reloaded.
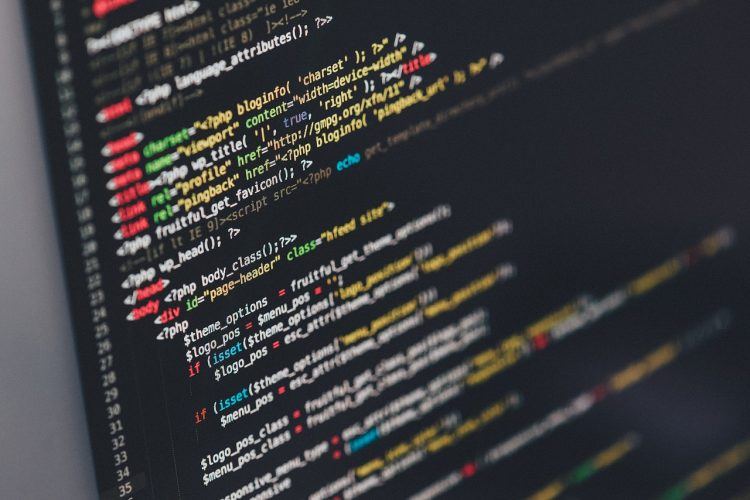
Android Testing with Kotlin.
In this post we will walk through how we can take advantage of the Kotlin Programming Language by introducing it in our codebase without affecting production code, in this case testing. This should be a good starting point towards a full migration. Continue reading Android Testing with Kotlin.
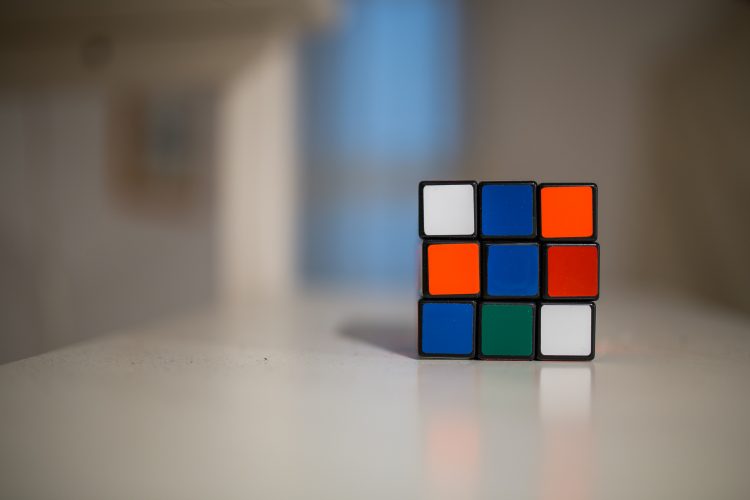
Android Architecture: Dynamic Parameters in Use Cases.
Your architectural approach should be adaptable enough to fulfill your requirements. In this article we will explore how we can convert our use cases to use dynamic parameters and gain more execution flexibility. Continue reading Android Architecture: Dynamic Parameters in Use Cases.
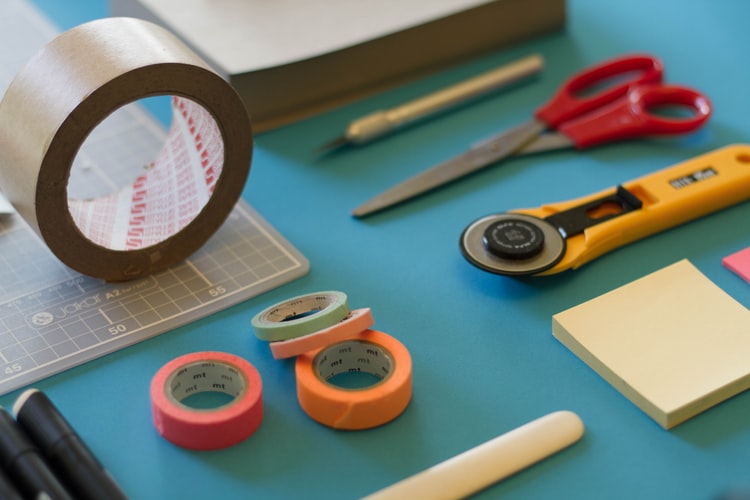
Android: Dagger 1 and 2 living together...
Migrating from one Dependency Injection Framework to another is not an easy task. Here, I present an approach to smooth the transition from Dagger 1 to Dagger 2 in a gradual way. Just know that we can have both versions of Dagger working together in Android. Continue reading Android: Dagger 1 and 2 living together...
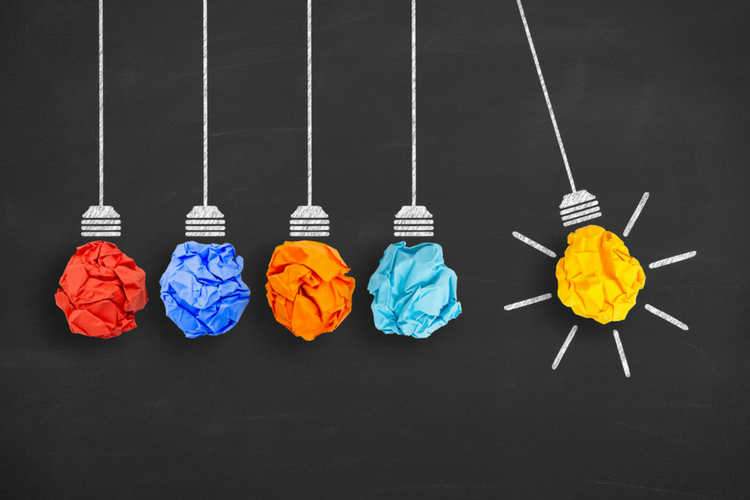
How to use Optional values on Java/Kotlin and Android
Optional values are very useful but unfortunately not available in Android (Java and Kotlin Standard Library) so in this post we will see how we can make use of them presenting different practical use case scenarios. Continue reading How to use Optional values on Java/Kotlin and Android
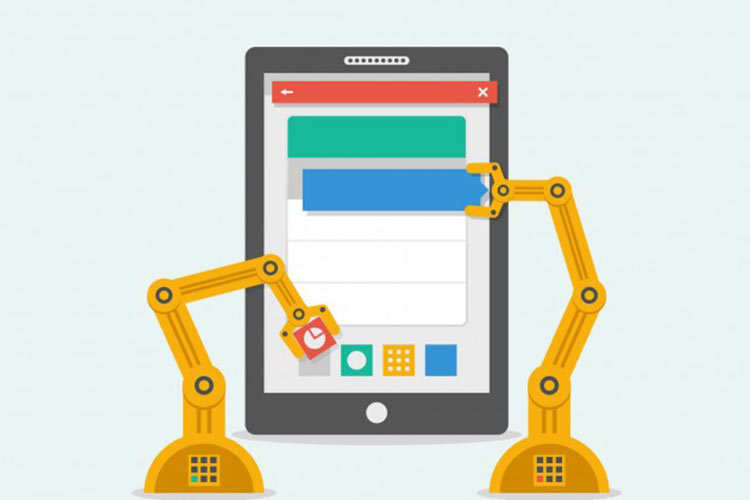
Debugging RxJava on Android.
Debugging is never and easy task but a very powerful way of finding to understand the code of finding the route cause of a bug. Here I will present an open source library called Frodo to debug RxJava asynchronous code. Continue reading Debugging RxJava on Android.
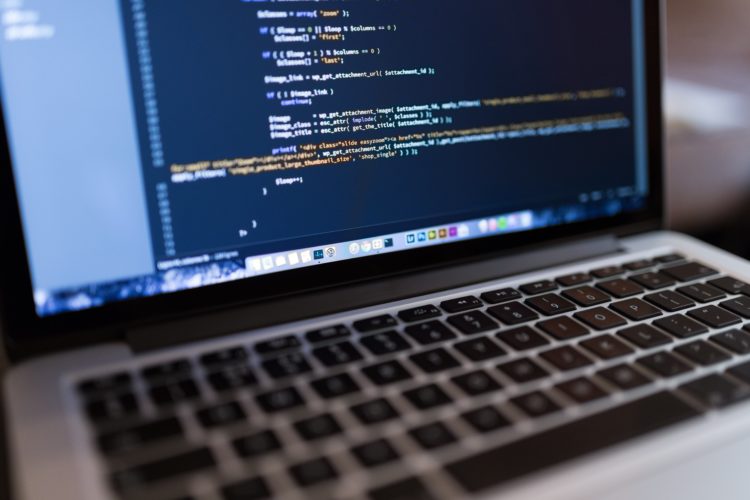
Architecting Android...The evolution.
Architecture is about evolution. Also on Android this is a constant work in progress, so in this post we will walk together through the evolution of my implementation of Clean Architecture on Android from previous posts. Continue reading Architecting Android...The evolution.
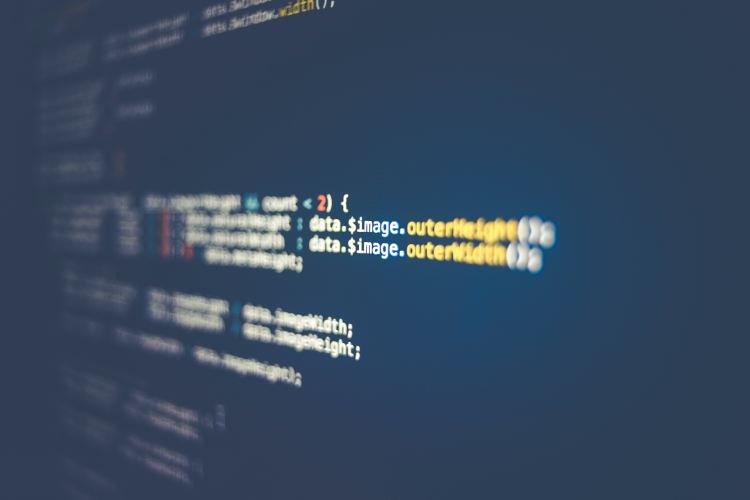
Tasting Dagger 2 on Android.
Dependency Injection (DI) is probably one of the most fundamental functionalities every software project should have. In this ocassion, we will see how Dagger 2 can help us and what is the logic behind its usage, in order to achieve objects injection in Android (OOP). Continue reading Tasting Dagger 2 on Android.
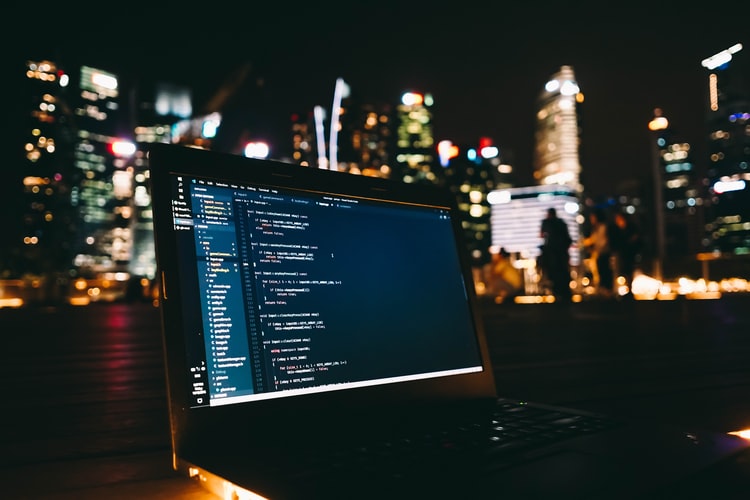
RxJava Observable tranformation: concatMap() vs flatMap().
There are so many operators which transform streams of data. Using the right ones could be challenging but something we ought to, so let’s clear up the main difference between concatmap() and flatmap() in RxJava, which can save us a bit of headache. Continue reading RxJava Observable tranformation: concatMap() vs flatMap().
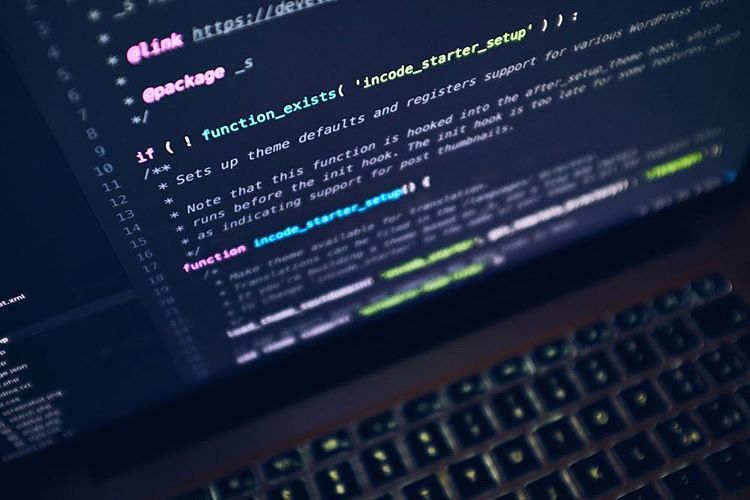
Architecting Android...The clean way?
Architecture is about evolution. The purpose of this article is to present an approach based on Clean Architecture from Robert C. Martin applied to Android. This is something I had in mind in the last few months, so let me share all the stuff I have learnt from investigating and implementing this pattern. Continue reading Architecting Android...The clean way?
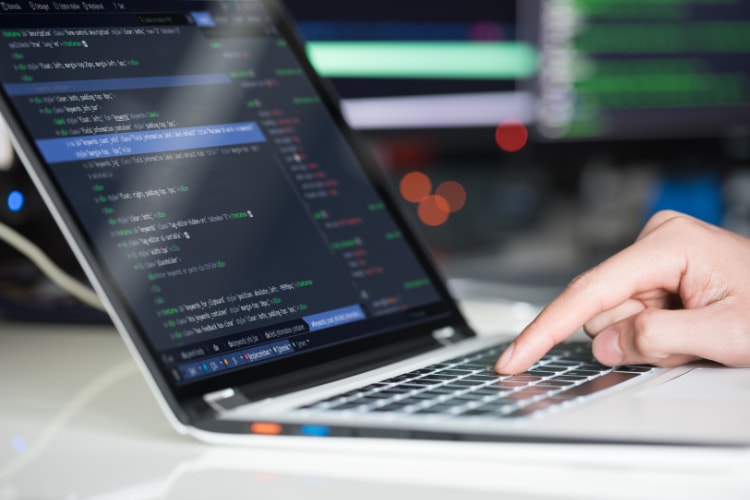
Aspect Oriented Programming in Android.
AOP (Aspect Oriented Programming) is a paradigm that has been with us for many years, and I found it very useful to apply it to Android. After some investigation, I consider that we can get a lot of advantages and very useful stuff when making use of it. Let’s get started! Continue reading Aspect Oriented Programming in Android.
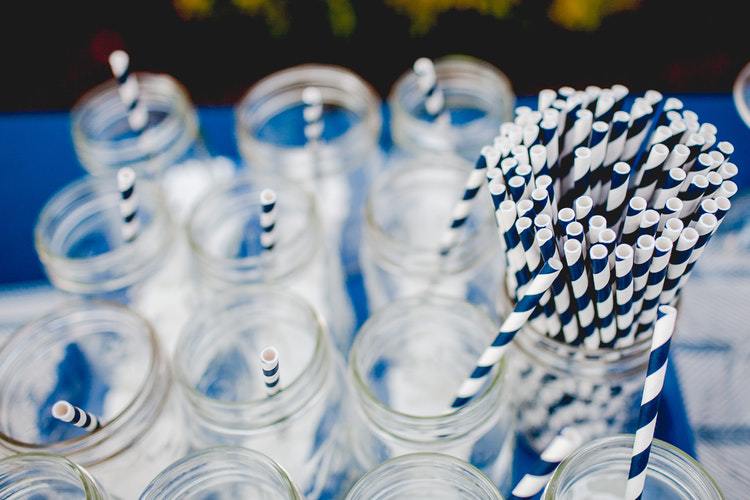
Unit testing asynchronous methods with Mockito.
Testing asynchronous code is one of the biggest challenges we have as engineers. There are tools out there, which facilitate our job, so in this opportunity, let’s explore how a Framework like Mockito can help us to achieve this goal. Continue reading Unit testing asynchronous methods with Mockito.